Lesson 01 - Creating the project
- How to create an MVC project
- Understand the pattern and relationship between controllers, action methods, and views.
The MVC template
I'll be using Visual Studio 2022. Open it up and select "Create New Project".
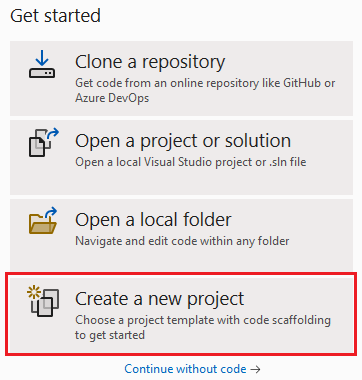
Select ASP.NET Core Web App (Model-View-Controller).
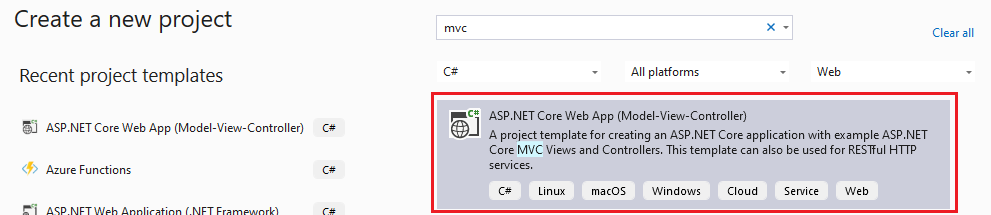
Choose a project name and a folder. I'm calling it "mvc-dotnet-shops".
Next, go ahead and choose the version of .NET you want. I'll use the latest with long term support which is .NET 8.0. The other options can be left on the defaults.

Visual Studio will generate your project with the standard MVC template. When it's finished, you will see folders like this in Solution Explorer:
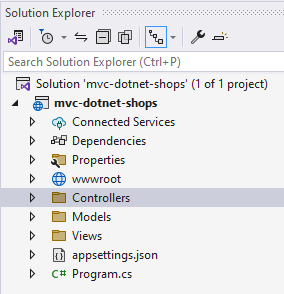
A solution is like a container for projects. We could put our app into different layers (for the popular "Clean Architecture" design), which would utilize multiple projects in a single solution. But, we'll stick with just the one. Clean Architecture is important because it's so common, but juniors aren't expected to fully understand it.
Our entire app will live inside one project — the one we already created. Notice the folders for Models, Views, and Controllers. One of the great things about MVC is that there are some standard structures and conventional ways of doing things. These standards and conventions help keep a codebase from getting too out of control. The other files we'll look at in upcoming lessons.
Start the app by clicking the button at the top with the filled-in green arrow. This button runs the app in debug mode. I typically choose the IIS Express option, but Visual Studio will set up local development servers for you no matter which you choose (I think).
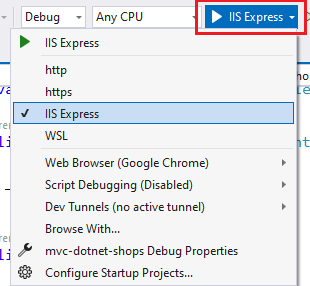
Things will happen under the hood, a browser will launch, and you'll see the default homepage of the template which probably looks like this:
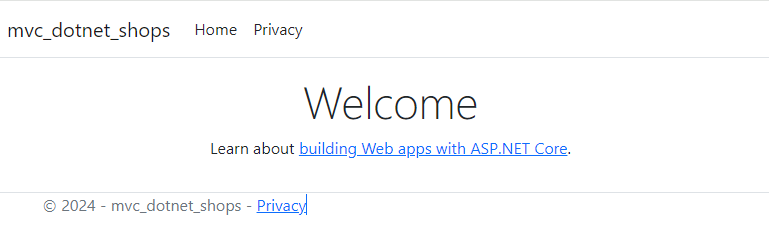
In your Solution Explorer, expand the Views folder, Home, and open up Index.cshtml.
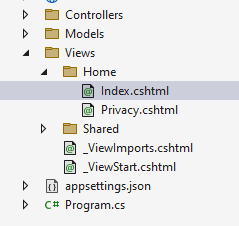
Make a change to the html, press ctrl + s (i.e. save the file), and you should see your change in the browser.
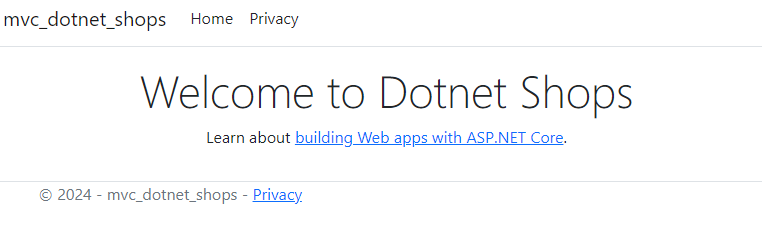
Note: Microsoft's hot reload can often be hit or miss. It's pretty good with MVC though. If your changes don't include any C# in your .cshtml file, you should be able to save and see the changes. If it's not working, just restart the app.
The 'V' and 'C' of MVC (views and controllers)
Now, expand the Controllers folder and open up HomeController.cs. Find the method named Index. It will look like this:
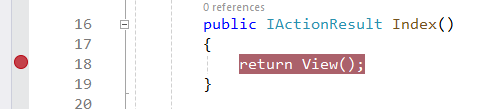
Put a breakpoint in it like I did above. The code will stop before View()
is returned. If your app is running, you can just refresh the page. Otherwise, run the app.
You'll hit your breakpoint before the page loads in the browser.
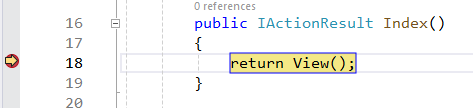
The key thing to note here is how the framework connects the controllers to the views in the Views folder.
Our HomeController correlates to the folder Views > Home.

Further, the Index
action method correlates the Index.cshtml file.
So, our Home controller, Index action method returns the Index.cshtml view located in the Views\Home folder.
More examples
Go back to the UI and click on the link to the Privacy page in the navbar.

It takes you to the privacy page. Notice the path in the URL: /Home/Privacy. Can you find the code in a controller that returns this view? Put a breakpoint where you think it might be and try to hit it.
Hover to see answer.
Because the path is /Home/Privacy, we can go to the Home controller and find the Privacy method. We simply need a breakpoint here:

What about the view? Can you find the .cshtml file, make a change, and see it on the Privacy page? Try it.
Hover to see answer.
In the Views folder, there's a Home folder, which has Privacy.cshtml. You can make any change and see it in the browser like this:
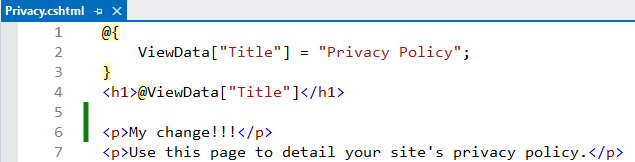
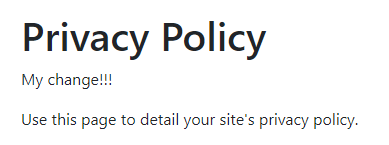
This pattern and relationship between controller, action method, and view is one of the most important aspects of the ASP.NET MVC framework. It makes the framework very nice and easy to use.